There are four ways to remove duplicate elements from a list in Kotlin using existing functions. In this article, we will learn about all of them. I also have an article on how to remove duplicates from an array in Kotlin that you can read here.
They all involve the use of an extension function on Kotlin Collection(s) classes in the Standard Template Library
- Using the `toSet()` extension function
- Using the `toHashSet()` extension function
- Using the `toMutableSet()` extension function
- Using the `distinct()` extension function
Consider the following example
You have a `data` class called `Vertex` represented as
data class Vertex(val id: Int, val description: String)
Now you have a list of `Vertex` objects represented as
val vertices = listOf(
Vertex(1, "remove duplicates from kotlin using toSet()"),
Vertex(2, "remove duplicates from kotlin using toHashSet()"),
Vertex(3, "remove duplicates from kotlin using distinct()"),
Vertex(4, "remove duplicates from kotlin using toMutableSet()"),
Vertex(5, "droidchef, android, gradle, kotlin")
)
If you encounter a `List` in Kotlin with duplicate elements, you will find solutions to all the questions mentioned below
- How to dedupe a list in Kotlin?
- How to remove duplicate elements from the list?
- How to find unique elements in the list?
- How to remove all the duplicates from a list in Kotlin?
Remove duplicates using toSet()
The first method of removing duplicates in Kotlin collection uses a process that converts the `List` into a new `Set`.
A Set is a data structure that by design does not allow duplicate elements. By converting a `List` into a `Set` you can easily remove duplicate elements. This is also the simplest and the best way to remove duplicates.
Points To Remember
- Return type of `toSet()` function is a `Set<T>`
- Internally it calls `toCollection()` function
- It guarantees the preservation of the original order of the elements.
- The returned `Set<T>` object is not mutable and only provides read access to the elements.
Sample Code
data class Vertex(val id: Int, val description: String)
val vertices = listOf(
Vertex(1, "android"),
Vertex(2, "gradle"),
Vertex(2, "gradle"),
Vertex(4, "jetpack compose"),
Vertex(5, "kotlin multiplatform")
)
val uniqueVertices = vertices.toSet()
uniqueVertices.forEach {
println(it)
}
Output
Vertex(id=1, description=android)
Vertex(id=2, description=gradle)
Vertex(id=4, description=jetpack compose)
Vertex(id=5, description=kotlin multiplatform)
Remove duplicates using toHashSet()
This function will create a new `HashSet` from the given `List`. A HashSet is an implementation of a `MutableSet` inteface.
Points To Remember
- Return type of `toHashSet()` function is a `HashSet<T>`
- Internally it calls `toCollection()` function
- It does not guarantee the preservation of the original order of the elements.
- The returned `HashSet<T>` object is mutable.
Code Example
data class Vertex(val id: Int, val description: String)
val vertices = listOf(
Vertex(1, "android"),
Vertex(2, "gradle"),
Vertex(2, "gradle"),
Vertex(4, "jetpack compose"),
Vertex(5, "kotlin multiplatform")
)
val uniqueVertices = vertices.toHashSet()
uniqueVertices.forEach {
println(it)
}
Output
Vertex(id=1, description=android)
Vertex(id=2, description=gradle)
Vertex(id=4, description=jetpack compose)
Vertex(id=5, description=kotlin multiplatform)
As you can see in the output, the elements are distinct and no duplicate element was printed.
Remove duplicates using distinct()
This function will return a new `List` that will not contain any duplicate elements from the original `List`. While it is the most flexible way to remove duplicates, as you can still use the original `List` if you need to. It is not the most efficient one.
Points To Remember
- Return type of `distinct()` is a `List`
- Internally it calls `toMutableSet()` function followed by `toList()` function
- Only use this if you want your return type to be a `List`.
- It guarantees the preservation of the original order of the elements.
- It is not an in place filtering function.
Code Example
data class Vertex(val id: Int, val description: String)
val vertices = listOf(
Vertex(1, "android"),
Vertex(2, "gradle"),
Vertex(2, "gradle"),
Vertex(4, "jetpack compose"),
Vertex(5, "kotlin multiplatform")
)
val uniqueVertices = vertices.distinct()
uniqueVertices.forEach {
println(it)
}
Output
Vertex(id=1, description=android)
Vertex(id=2, description=gradle)
Vertex(id=4, description=jetpack compose)
Vertex(id=5, description=kotlin multiplatform)
Remove duplicates using toMutableSet()
This function will create a new `MutableSet` from the given List.
Points To Remember
- Return type of `toMutableSet()` function is a `MutableSet<T>`
- Internally it calls `toCollection()` function
- It preserves the original order of the elements as it uses `LinkedHashSet<T>` implementation of a `MutableSet<T>`
Code Example
data class Vertex(val id: Int, val description: String)
val vertices = listOf(
Vertex(1, "android"),
Vertex(2, "gradle"),
Vertex(2, "gradle"),
Vertex(4, "jetpack compose"),
Vertex(5, "kotlin multiplatform")
)
val uniqueVertices = vertices.toMutableSet()
uniqueVertices.forEach {
println(it)
}
Output
Vertex(id=1, description=android)
Vertex(id=2, description=gradle)
Vertex(id=4, description=jetpack compose)
Vertex(id=5, description=kotlin multiplatform)
Remove Duplicates using a Loop Manually
If you want to remove duplicate elements from a List or Kotlin collections manually.
Here is an example code to iterate over the list and manually filter out the distinct items from the return list.
This is not the top suggested approach as the time complexity could potentially increase when writing this piece of code and performing all these operations, especially on a resource-constrained system.
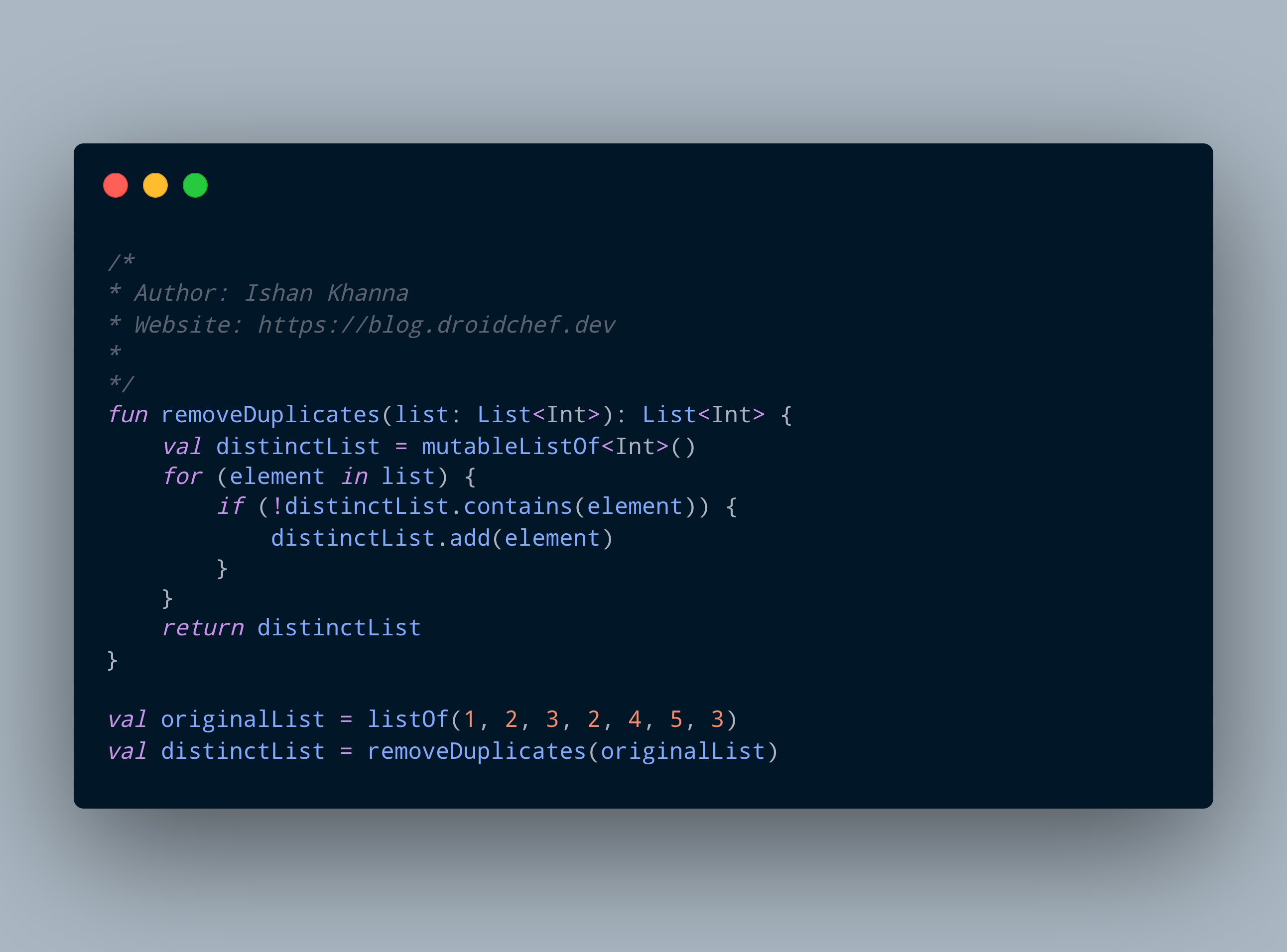
Output
[1, 2, 3, 4, 5]
The removeDuplicates function takes a List<Int> as its parameter and returns a new list with duplicates removed.
Inside the function, we define a mutableListOf to store the distinct elements, and use a for-loop to iterate through each element in the original list. For each element, we check if it already exists in the distinctList using the contains() function.
If it doesn't, we add it to the distinctList. Finally, we return the distinctList as the result.
Conclusion
There are a few different ways to remove duplicates from a List in kotlin, each with its own benefits and drawbacks.
You can use functions from the standard template library provided by Kotlin remove duplicates from list.
You can also write your own `foreach` loop and go over each index to filter out duplicate instance of elements in your collections.
The best way to choose which method to use depends on your specific needs. If you're working with a large list, you need to make sure that the method you're using is efficient enough to handle it.
Frequently Asked Questions
Q: What is the best way to remove duplicates from a List in Kotlin?
Answer - The best way to remove duplicates from a List in Kotlin is to use the `toSet()` method if you don't mind working with a `Set<T>` as it also preserves the original order of elements. If you don't care about the order and only want to have a `List<T>` as the return type then you should use `distinct()` function.
Q: What are some of the challenges people face when trying to remove duplicates from a List?
Answer - Some of the challenges people face when trying to remove duplicates from a List include not knowing which method to use, and its efficiency in terms of time complexity and space complexity. Another important thing to consider is whether the duplicates are being removed in place or not. If you're working with a large list, you need to make sure that the method you're using is efficient enough to handle it.
Q: What is the most efficient way to remove duplicates from a List in Kotlin?
Answer- The most efficient way to remove duplicates from a List in kotlin is to use the `toSetOf()` function. This function will create a new Set<T> (which is a data structure that doesn't allow duplicate entries) from the given list. It uses a hashing algorithm to store the elements in the `Set`, so it's very efficient.
Learn in-depth about Gradle Dependency Trees and Five Ways to Generate Gradle Dependency Tree.
Subscribe to my blog and never miss an update on new articles about Android and Gradle.